Why Python?
This crash course will teach you the basics of the Python programming language.
Motivation
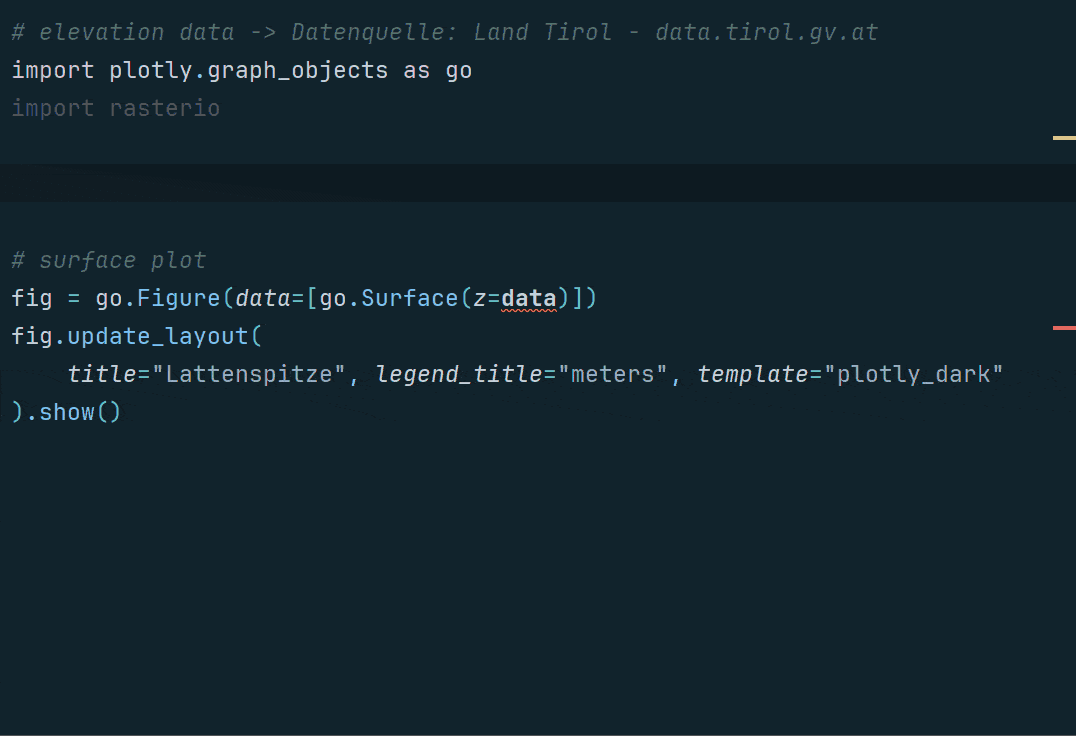
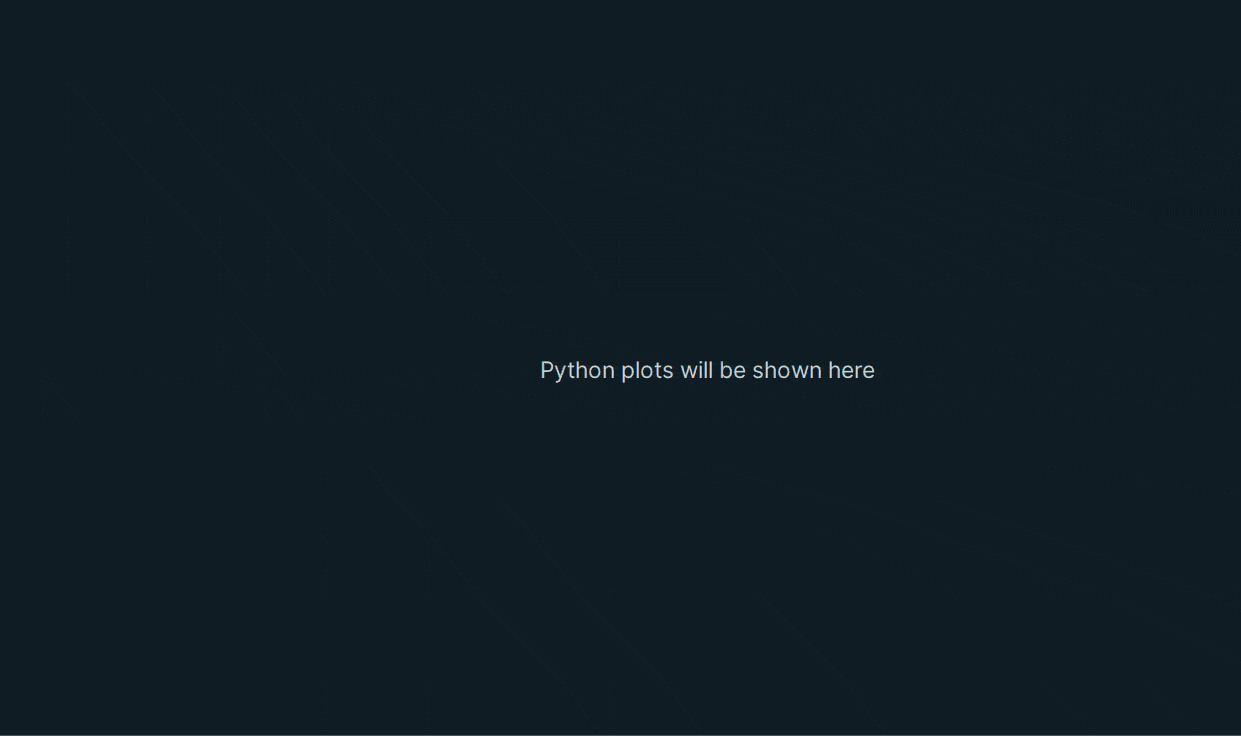
That's the power of Python - ease of use paired with a wide range of functionalities stemming from a large developer community! 🦾
-
Ease of use
Python
with its syntax is easy to learn and yet very powerful. -
Flexible
Python
is a versatile language that can be used for web development, data analysis, artificial intelligence, and many more.
The below section should give you an impression of what you can do with
Python
. This is not an extensive list by all means. It might sound
trashy but if you can imagine something you probably can build it in
Python
.
Don't worry about the code snippets too much, after finishing the course you'll have a better understanding of them and will be able to run and modify code yourself. For now, the snippets illustrate the capabilities of the language and what complex things you can achieve with little code.
Examples
Visualizations
You can create stunning and interactive visualizations1.
# Source: https://plotly.com/python/tile-county-choropleth/
import plotly.express as px
df = px.data.election()
geojson = px.data.election_geojson()
fig = px.choropleth_map(
df,
geojson=geojson,
color="Bergeron",
locations="district",
featureidkey="properties.district",
center={"lat": 45.5517, "lon": -73.7073},
map_style="carto-positron",
zoom=9,
)
fig.show()
Machine Learning/AI
... or you can easily train your own machine learning models. In this example, with just a few lines of code, a decision tree is fit and visualized2.
# Source: https://scikit-learn.org/stable/auto_examples/tree/plot_unveil_tree_structure.html#train-tree-classifier
import matplotlib.pyplot as plt
from sklearn.datasets import load_iris
from sklearn.model_selection import train_test_split
from sklearn.tree import DecisionTreeClassifier, plot_tree
iris = load_iris()
X = iris.data
y = iris.target
X_train, X_test, y_train, y_test = train_test_split(X, y, random_state=0)
clf = DecisionTreeClassifier(max_leaf_nodes=3, random_state=0)
clf.fit(X_train, y_train)
plot_tree(clf)
plt.savefig("tree.svg")
Automation
... or automate tasks that you would have otherwise done manually. This code snippet fetches some data (from an online service) and writes it to a file3.
import json
from pathlib import Path
import httpx
url = "https://pokeapi.co/api/v2/pokemon/charmander"
response = httpx.get(url)
with Path("charmander.json").open("w") as file:
json.dump(response.json(), file, indent=4)
Web Development
You can create websites, just like this one. In fact, all the
heavy lifting of this site is done by Python
and tools developed by its
community.
Getting Started...
In the next sections, we will install Python
including the code editor
Visual Studio Code
.
Info
Both Python and Visual Studio Code are already pre-installed on PCs in the MCI computer rooms. If you are working with your own computer, please proceed to the installation page.