More control structures
Introduction
In this section, we will cover additional control structures. First, we
discuss the if
statement, which allows us to execute code based on a
condition. Followed by the elif
, else
and
while
statements.
if
The if
statement lets you evaluate conditions. The simplest kind of
if
statement has one condition and one action. Here is some
pseudocode:
You can put any condition in the first line and just about any action in the
indented block following the test. If the condition evaluates to True
,
Python
executes the indented code following the if
statement.
If the test evaluates to False
, the indented code block (following the
if
) is ignored.
First, the condition user == "admin"
is evaluated. If it
evaluates to True
, the indented print is executed. If the condition
evaluates to False
, the indented code block is ignored.
Indentation plays the same role in if
statements as it did in
for
loops (see the previous section).
Password strength: Part 1
In the section on comparisons and logical operators, you had to check whether a password meets certain criterias. The following example expands on this task as you are given a list of passwords. You have to check if each password exceeds a length of 12 characters.
Execute the first code cell to generate some random passwords (note every time you rerun the code snippet, different passwords will be generated).
# generate passwords - simply execute the code to generate some random
# passwords
import random
import string
passwords = []
for i in range(10):
length = random.randint(3, 25)
password = "".join(random.choices(string.ascii_letters, k=length))
passwords.append(password)
The list
passwords
should look something like this:
['PWgOYxQgnxgXm',
'gpOMVTmCSjAcndowkUd',
'ADKIEthzsGBr',
'VRLzOIZtEz',
'uOckmTJjeonUyMlnG',
'gjOpWuHrIbG',
'doxIylbRkNLdvdLNgVgYsDGzd',
'KvUdsgZhPIrS',
'LrdpffEqlBVQYr',
'ncyqXNLnVstVxlx']
Now, loop over the passwords and check if each password exceeds the character limit of 12. If so, print the password.
else
Previously, every time the condition in the if
statement
evaluated to False
,
no action was taken. Hence, the else
clause is introduced which
allows you to define a set of actions that are executed when the conditional
test fails.
user = "random_user"
if user == "admin":
print(f"Welcome {user}!")
else:
print("Only admins can enter this area!")
Password strength: Part 2
Let's expand on our previous example. Re-use your code to check the length
of the generated passwords. Now, we would like to store all passwords that
did not meet our criteria in the empty list invalid_passwords
.
Hint: Introduce an else
statement to save the invalid passwords.
elif
Often, you’ll need to test more than two possible situations, and to evaluate
these, you can use an if-elif-else
syntax. Python
executes only one
block in an if-elif-else
chain. It runs each conditional test in order until
one passes. When a test passes, the code following that test is executed and
the rest is skipped.
user = "xX_user_Xx"
registered_users = [
"admin",
"guest",
"SwiftShark22",
"FierceFalcon66",
"BraveWolf11"
]
if user == "admin":
print(f"Welcome {user}!")
elif user not in registered_users:
print("Please create an account first!")
else:
print("Only admins can enter this area!")
Info
As you might have noticed, you can use a single if
statement or
if
in combination with else
. For multiple conditions
you can add as many elif
parts as you wish.
while
The for
loop takes an iterable and executes a block of
code once for each element. In contrast, the while
loop runs as
long as a certain condition is True
.
For instance, you can use a while
loop to count up through a
series of numbers. Here is an example:
# set a counter
current_number = 1
while current_number <= 5:
print(current_number)
# increment the counter value by one
current_number += 1
Note, that the variable, that is checked in the while
-condition
must be defined prior to the loop, otherwise we will encounter a
NameError
.
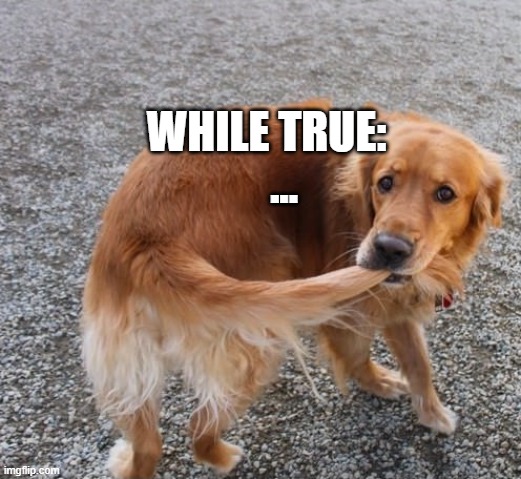
Moreover, the variable must be updated within the loop
to avoid an infinite loop. For example, if current_number
is not
incremented by one, the condition current_number <= 5
will always
evaluate to True
, leaving us stuck in an infinite loop.
In such cases, simply click the Stop
button (on the left-hand side of the
respective code cell) to interrupt the execution.
Addition assignment
In the above example, we used the +=
operator, referred to as
addition assignment. It is a shorthand for incrementing a variable by a
certain value.
The above code is equivalent to a = a + 5
.
This shorthand assignment can be used with all arithmetic
operators, such as subtraction -=
or division /=
.
While loop
Write some code, to print all even numbers up to 42 using a while loop.
Detour: User input
Most programs are written to solve an end user’s problem. To do so, usually we need to get some information from the user. For a simple example, let’s say someone wants to enter a username.
You can store the user input in the variable user_name
like in the example
below.
However, the input()
function always returns a string.
... use casting to convert the input to the desired type.
break
To exit any loop immediately without running any remaining 'loop code', use
the break
statement. The break
statement directs the flow
of your program; you can use it to control which lines of code are executed and
which aren’t, so the program only executes code that you want it to, when you
want it to.
continue
Rather than breaking out of a loop entirely, you can use the continue
statement to return to the beginning of the loop based on the result of a
condition.
Recap
In this section we have expanded on control structures. We discussed:
if
statements and how to use themelse
clauseselif
statements for multiple conditionswhile
loopsbreak
andcontinue
statements for more 'fine-grained' control